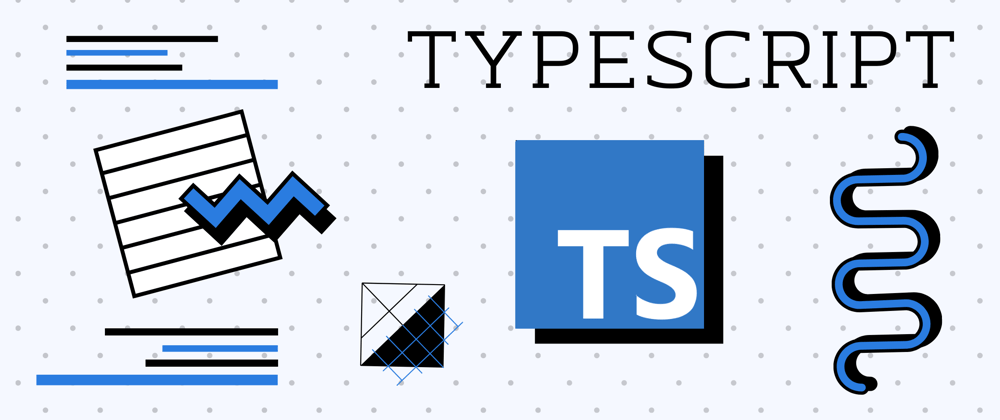
Fri Jan 05 - Written by: Ritesh Kumar
NodeJs Typescript Starter
How To Make A Typescript + NodeJS Express Project with eslint, EJS and Restart On Typescript Server Changes
Start with a clean project
pnpm init
or
npm init -y
Install some dependencies
pnpm add -save-dev eslint @types/node @types/express typescript ts-node-dev
pnpm add --save express ejs
or
npm install -save-dev eslint @types/node @types/express typescript ts-node-dev
npm install --save express ejs
make a new folder for your project
mkdir src
touch src/app.ts
src/app.ts
import express from 'express';
const app = express();
const port = 3000;
app.use(express.json());
app.use(express.static("public"));
app.set("view engine", "ejs");
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
return console.log(`http://localhost:${port}`);
});
create a new folder for your public folder
create a new folder of views
Create tsconfig.json
{
"compilerOptions": {
"module": "commonjs",
"esModuleInterop": true,
"target": "es6",
"moduleResolution": "node",
"sourceMap": true,
"outDir": "dist"
},
"lib": [
"es2015"
]
}
Now we will run eslint’s initialization command to interactively set up the project:
npx eslint --init
Now You have to answer some questions to finish the initialization process:
- How would you like to use ESLint?: To check syntax and find problems
- What type of modules does your project use?: JavaScript modules (import/export)
- Which framework does your project use?: None of these
- Does your project use TypeScript?: Yes
- Where does your code run?: Node
- What format do you want your config file to be in?: JavaScript
Finally, you will be prompted to install some additioanl eslint libraries. Choose Yes. The process will finish and you’ll be left with the following configuration file:
Now we will use ts-node-dev for watching the changes in our typescript server file
As we already installed the dev dependency we do not have to do much we just have to add the start script in our package.json file
Lets Change our package.json and add some lines
add main
"main": "dist/app.js",
add lint and start in scripts
"lint": "eslint . --ext .ts",
"start": "ts-node-dev src/app.ts"
Finally It should look like this
{
"name": "typescript-node",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"lint": "eslint . --ext .ts",
"start": "ts-node-dev src/app.ts"
},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"@types/express": "^4.17.13",
"@types/node": "^18.0.0",
"@typescript-eslint/eslint-plugin": "^5.30.0",
"@typescript-eslint/parser": "^5.30.0",
"eslint": "^8.18.0",
"ts-node-dev": "^2.0.0",
"typescript": "^4.7.4"
},
"dependencies": {
"ejs": "^3.1.8",
"express": "^4.18.1"
}
}
Now start the project with pnpm or npm
pnpm start
or
npm start
Hurray you have a new project! 🎉
Connect me on Twitter :- Twitter 🤝🏻
Do check out my Github for amazing projects:- Github 🤝🏻
Connect me on LinkedIn :- Linkedin 🤝🏻